Python Kubernetes Client: A Comprehensive Guide to Managing Kubernetes Clusters

Tasrie IT Services
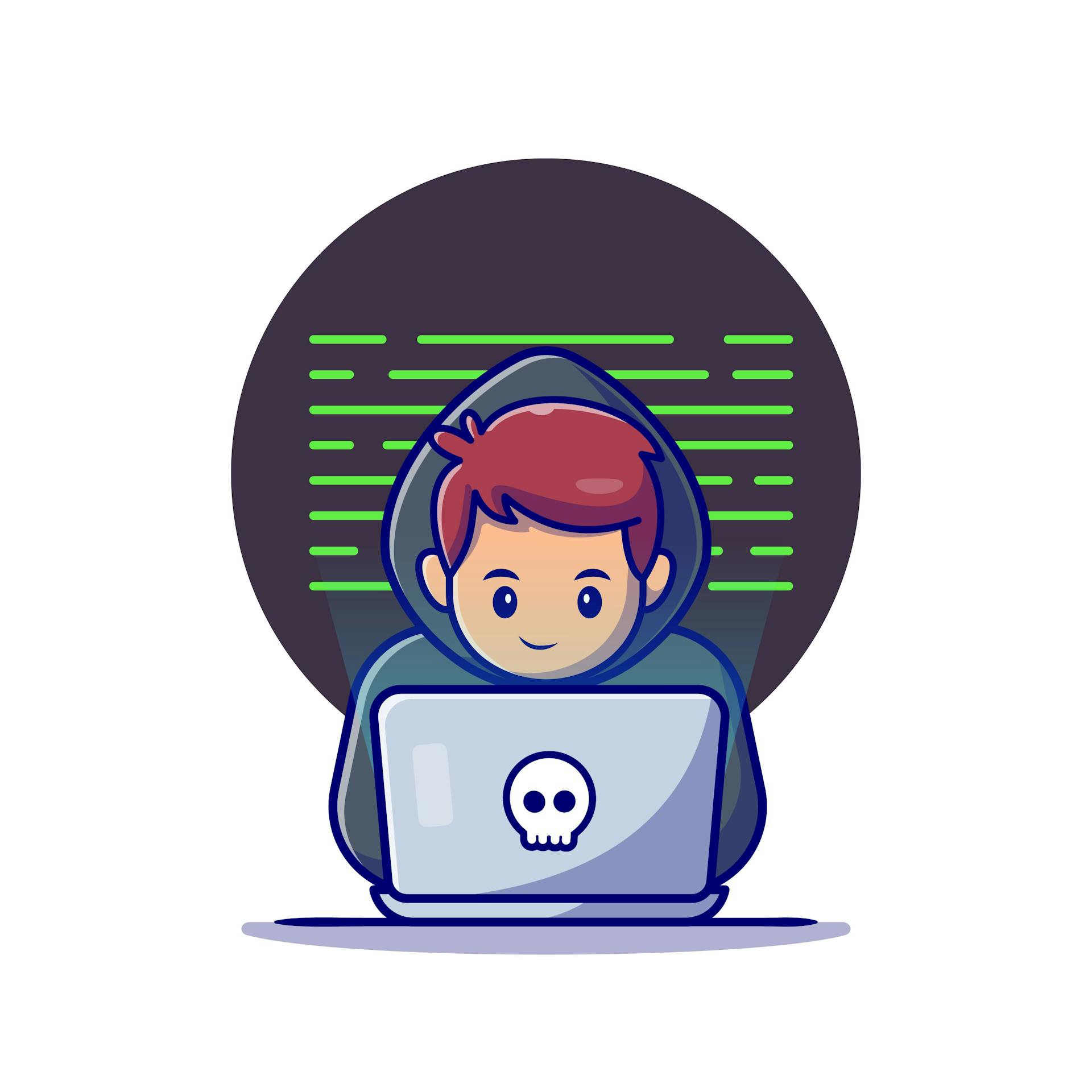
Introduction
Are you looking to streamline your Kubernetes cluster management tasks? Look no further! In this article, we will explore the Python Kubernetes Client, a powerful tool that allows you to interact with Kubernetes clusters seamlessly. Whether you are a seasoned Kubernetes expert or a newcomer to the world of container orchestration, this guide has got you covered. Let's dive in and discover how the Python Kubernetes Client can enhance your Kubernetes experience.
Python Kubernetes Client: Exploring the Basics
The Python Kubernetes Client is a library that provides Python bindings for the Kubernetes API. It allows users to interact with Kubernetes clusters programmatically, enabling automation, resource management, and monitoring of containerized applications. By leveraging the Python Kubernetes Client, you can efficiently manage clusters, deploy applications, and monitor their performance with ease.
Installation and Setup
Before we delve into the various capabilities of the Python Kubernetes Client, let's first set it up and get it running on your system. Follow these simple steps to install the client:
-
Open your terminal or command prompt.
-
Use the following pip command to install the Python Kubernetes Client:
pip install kubernetes
- Once the installation is complete, you can start using the Python Kubernetes Client to interact with Kubernetes clusters.
Authenticating with Kubernetes Clusters
To utilize the Python Kubernetes Client effectively, you need to authenticate with your Kubernetes clusters. There are several authentication methods supported, including:
-
Kubeconfig: The client reads credentials and cluster information from a kubeconfig file.
-
Service Account: If your code is running within a Kubernetes pod, you can use the service account's credentials for authentication.
-
Client Certificate: You can provide client certificate and key files for authentication.
Ensure you have the appropriate credentials and access permissions before proceeding.
Exploring Key Features of the Python Kubernetes Client
The Python Kubernetes Client comes with a plethora of features that simplify the management of Kubernetes clusters. Let's explore some of its key capabilities:
1. Resource Management
The Python Kubernetes Client allows you to create, read, update, and delete various Kubernetes resources programmatically. You can manage pods, deployments, services, config maps, and more, all from within your Python code.
Example:
# Create a new Deployment
import yaml
from kubernetes import client, config
config.load_kube_config()
api_instance = client.AppsV1Api()
deployment_manifest = yaml.safe_load("""
apiVersion: apps/v1
kind: Deployment
metadata:
name: nginx-deployment
spec:
replicas: 3
selector:
matchLabels:
app: nginx
template:
metadata:
labels:
app: nginx
spec:
containers:
- name: nginx
image: nginx:latest
""")
api_instance.create_namespaced_deployment(namespace="default", body=deployment_manifest)
2. Scalability and Auto-scaling
The Python Kubernetes Client facilitates scaling your applications effortlessly. You can dynamically adjust the number of replicas for deployments based on CPU utilization or custom metrics.
Example:
# Scale a Deployment
api_instance = client.AppsV1Api()
api_instance.patch_namespaced_deployment_scale(
name="nginx-deployment",
namespace="default",
body={"spec": {"replicas": 5}}
)
3. Pod Monitoring
With the Python Kubernetes Client, you can access real-time information about your pods, including their status, resource usage, and logs.
Example:
# Get pod information
api_instance = client.CoreV1Api()
pod_list = api_instance.list_namespaced_pod(namespace="default")
for pod in pod_list.items:
print(f"Name: {pod.metadata.name}, Status: {pod.status.phase}")
4. ConfigMap Management
ConfigMaps are an essential part of configuring applications in Kubernetes. The Python Kubernetes Client enables you to create, update, and delete ConfigMaps with ease.
Example:
# Create a new ConfigMap
config_map_manifest = yaml.safe_load("""
apiVersion: v1
kind: ConfigMap
metadata:
name: app-config
data:
key1: value1
key2: value2
""")
api_instance = client.CoreV1Api()
api_instance.create_namespaced_config_map(namespace="default", body=config_map_manifest)
Advanced Techniques with the Python Kubernetes Client
Now that we have covered the basics and explored some key features, let's dive into more advanced techniques using the Python Kubernetes Client.
5. Custom Resource Definitions (CRDs)
CRDs enable you to extend the Kubernetes API and define your custom resources. The Python Kubernetes Client supports CRD management, allowing you to create and interact with custom resources effortlessly.
Example:
# Create a custom resource
custom_resource_manifest = yaml.safe_load("""
apiVersion: apiextensions.k8s.io/v1
kind: CustomResourceDefinition
metadata:
name: mycrd.example.com
spec:
group: example.com
version: v1
names:
kind: MyCRD
singular: mycrd
plural: mycrds
""")
api_instance = client.ApiextensionsV1Api()
api_instance.create_custom_resource_definition(body=custom_resource_manifest)
6. Handling Persistent Volumes
Persistent Volumes (PVs) play a crucial role in Kubernetes storage management. The Python Kubernetes Client enables you to manage PVs and dynamically provision storage.
Example:
# Create a Persistent Volume Claim (PVC)
pvc_manifest = yaml.safe_load("""
apiVersion: v1
kind: PersistentVolumeClaim
metadata:
name: my-pvc
spec:
accessModes:
- ReadWriteOnce
resources:
requests:
storage: 1Gi
""")
api_instance = client.CoreV1Api()
api_instance.create_namespaced_persistent_volume_claim(namespace="default", body=pvc_manifest)
Best Practices for Using the Python Kubernetes Client
To make the most of the Python Kubernetes Client, keep these best practices in mind:
7. Error Handling
Always implement proper error handling when interacting with Kubernetes clusters. Handle potential exceptions and failures gracefully to ensure smooth execution of your code.
8. Version Compatibility
Ensure that your Python Kubernetes Client version aligns with the Kubernetes version running on your clusters. Mismatched versions can lead to unexpected behaviors.
9. Resource Cleanup
When creating resources programmatically, remember to clean up unused or temporary resources to avoid cluttering your cluster.
FAQs
-
Can I use the Python Kubernetes Client with any Kubernetes distribution? Yes, the Python Kubernetes Client is compatible with any Kubernetes distribution, whether it's self-hosted or managed Kubernetes services like Google Kubernetes Engine (GKE) or Amazon Elastic Kubernetes Service (EKS).
-
Is the Python Kubernetes Client officially supported by Kubernetes? While the Python Kubernetes Client is a community-driven project, it is widely adopted and supported by the Kubernetes community. It is considered a reliable tool for interacting with Kubernetes clusters.
-
Does the Python Kubernetes Client work with both Python 2 and Python 3? The Python Kubernetes Client primarily supports Python 3. Python 2 reached its end-of-life on January 1, 2020, and it is recommended to use Python 3 for all projects.
-
Can I use the Python Kubernetes Client for managing multiple Kubernetes clusters? Absolutely! The Python Kubernetes Client allows you to manage multiple clusters with ease. You can switch between contexts and interact with different clusters from a single Python script.
-
Is the Python Kubernetes Client suitable for managing production-grade clusters? Yes, the Python Kubernetes Client is a powerful tool suitable for managing production-grade Kubernetes clusters. However, as with any tool, it is crucial to exercise caution and follow best practices to ensure the stability and reliability of your clusters.
-
Can I extend the Python Kubernetes Client's functionality with plugins? While the Python Kubernetes Client provides a comprehensive set of features, you can extend its functionality by integrating custom plugins or contributing to the open-source project.
Conclusion
In conclusion, the Python Kubernetes Client is a valuable asset for anyone working with Kubernetes clusters. Its intuitive Python bindings and rich feature set make managing clusters, deploying applications, and monitoring resources a breeze. By using the Python Kubernetes Client, you can unlock the full potential of Kubernetes and enhance your container orchestration experience.
So, what are you waiting for? Embrace the power of the Python Kubernetes Client and take your Kubernetes management to new heights!
============================================
We provide DevOps Consulting services, make sure to check out the page
For more such content, make sure to check out our latest tech blog
Follow our LinkedIn Page