Creating a Simple Microservice in Java Spring: A Step-by-Step Guide

Tasrie IT Services
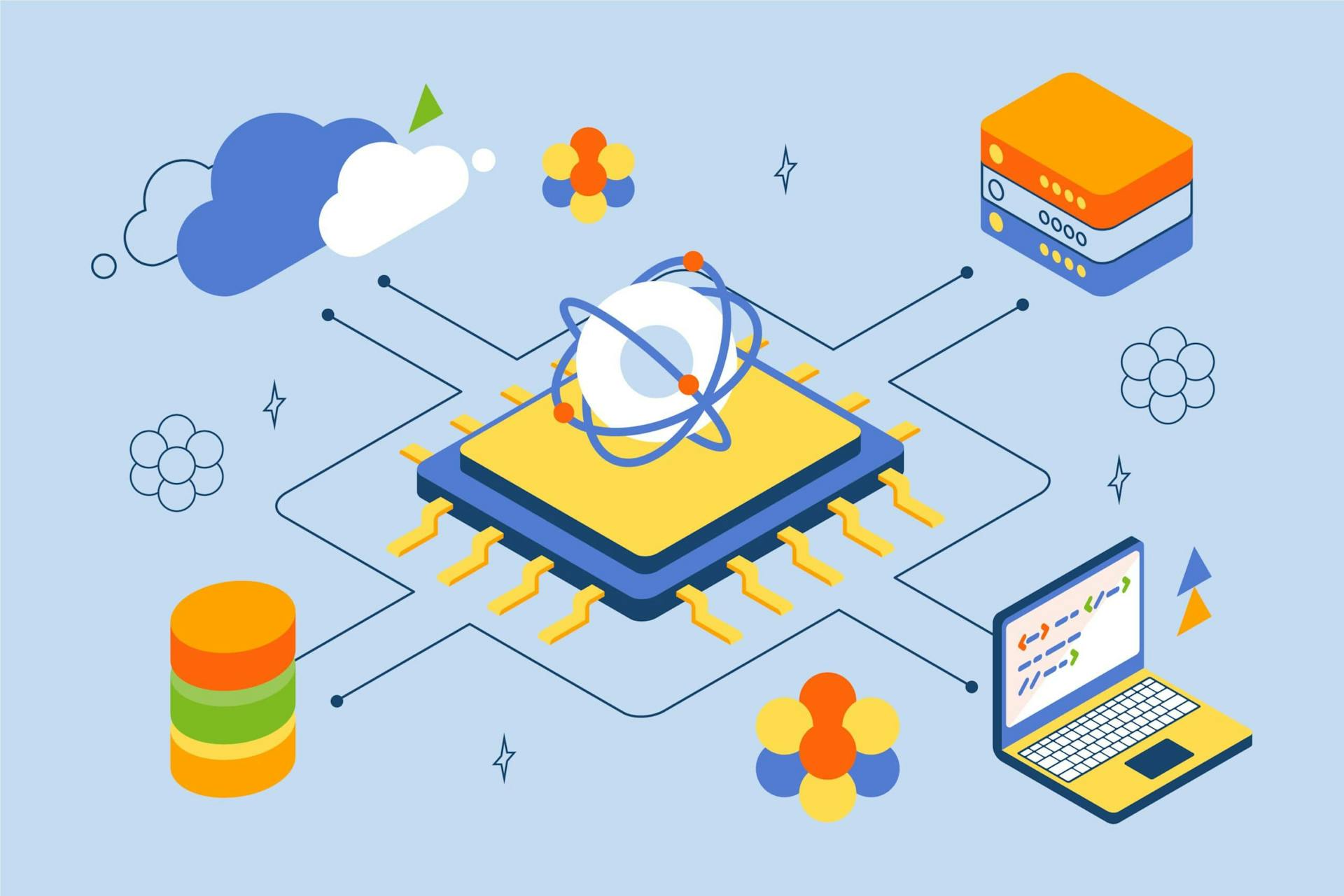
Image by Freepik
Creating a microservice in Java Spring is a great way to build scalable and maintainable applications. In this post, we will cover the basic steps involved in creating a simple microservice in Java Spring.
Setup Spring Boot
The first step is to set up the Spring Boot framework, which will make it easy to create and manage our microservice. To do this, we need to create a new project in your preferred IDE and add the necessary dependencies for Spring Boot.
In IntelliJ IDEA, for example, you can create a new Spring Boot project by selecting "New Project" from the File menu and choosing "Spring Initializr" as the project type. Then, you can add the following dependencies:
Spring Web
Spring Boot DevTools
After adding the dependencies, you can click "Finish" to create the project.
Define the REST Endpoint
Once the project is set up, we can start defining the REST endpoint for our microservice. This can be done by creating a new Java class and annotating it with the @RestController
annotation.
For example, let's create a class called GreetingController
:
@RestController
public class GreetingController {
@GetMapping("/hello")
public String sayHello() {
return "Hello, World!";
}
}
In this example, we define a GET endpoint /hello
that returns the string "Hello, World!".
Step 3: Build and Run the Microservice
With the REST endpoint defined, we can now build and run the microservice. This can be done using the command line or through your IDE's built-in tools.
If you prefer the command line, you can use the following commands to build and run the microservice:
./mvnw clean package
java -jar target/my-microservice.jar
If you're using an IDE, you can usually run the microservice by right-clicking on the main class and selecting "Run".
Step 4: Test the Microservice
Finally, we can test the microservice to make sure it's working as expected. We can do this by making a request to the /hello
endpoint using a tool like Postman or cURL.
For example, if we're running the microservice on our local machine, we can make a GET request to
http://localhost:8080/hello:
GET http://localhost:8080/hello
If everything is working correctly, we should receive a response containing the string "Hello, World!".
And that's it! We've successfully created a simple microservice in Java Spring. Of course, this is just a basic example, and there are many more features and best practices to consider when building microservices. But this should give you a good starting point for building your own microservices using Java Spring.